In the world of modern software development, Model-View-Controller (MVC) architecture is a staple for building dynamic and organized web applications. While many guides focus heavily on GUI-based tools and IDEs, building an MVC web app from the terminal can deepen your understanding of the underlying processes. This step-by-step guide will take you through creating a basic MVC web application entirely from the terminal, using Node.js and Express as the foundation.
Table of Contents
1. Setting Up Your Development Environment
Ensure you have the tools needed for this project:
- Node.js installed on your system. You can use
node -v
to check the current version. - NPM (Node Package Manager), which usually comes bundled with Node.js.
- A text editor, such as Vim, Nano, or any terminal-based editor.
Open your terminal and navigate to the directory where you’d like to build your app. Run the following command to create a new project:
mkdir mvc-web-app && cd mvc-web-app
Next, initialize a Node.js project:
npm init -y
This will generate a package.json
file, which will track dependencies and project details.
2. Installing Dependencies
For our application, we need Express (a Node.js web framework) and a view engine like EJS. Install these with:
npm install express ejs
You may also want to install the nodemon package, which reloads your app automatically when you make changes:
npm install --save-dev nodemon
Your project structure should now contain a node_modules
folder, package.json
, and a package-lock.json
file.
3. Structuring the MVC Folders
To organize the application following the MVC pattern, create the necessary directories:
mkdir controllers models views public
Each folder serves a distinct purpose:
- controllers/: Handles business logic and routes.
- models/: Manages data and database interactions.
- views/: Contains templates for rendering the frontend.
- public/: Stores static assets like CSS, JS, and images.
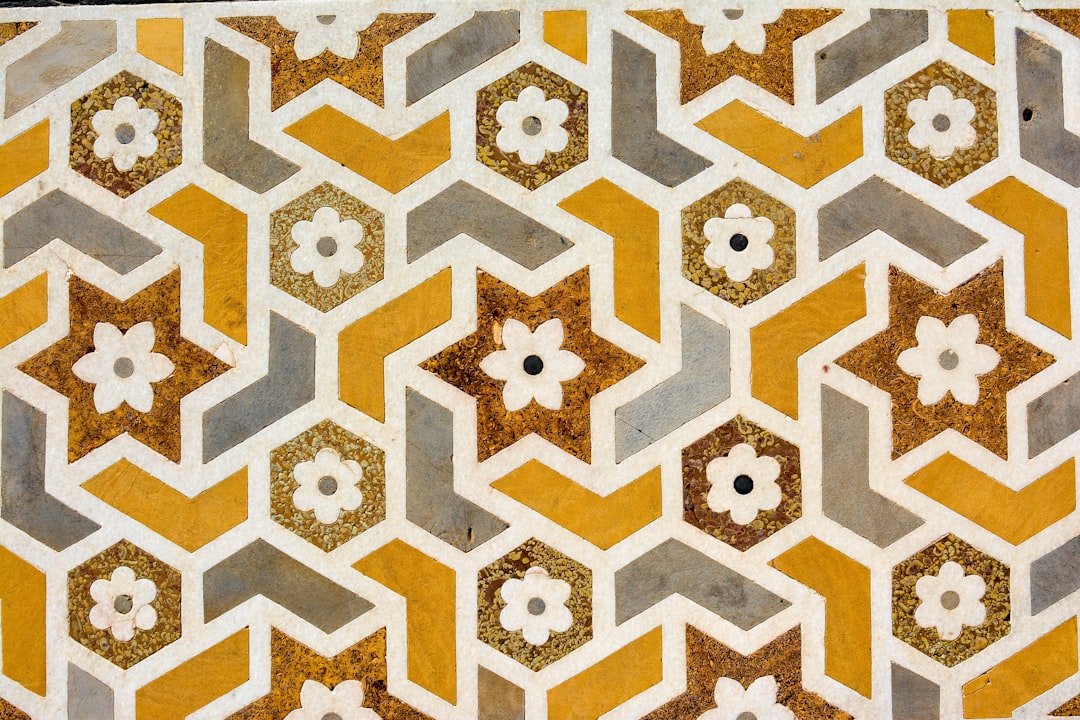
4. Creating a Simple Server
Create a new file named index.js
in the root folder of your project. This file will act as the entry point for your application. Open the file in a terminal-based editor and insert the following code:
const express = require('express');
const app = express();
const port = 3000;
// Set EJS as the view engine
app.set('view engine', 'ejs');
// Routes
app.get('/', (req, res) => {
res.render('index', { message: 'Welcome to the MVC Web App!' });
});
// Start the server
app.listen(port, () => {
console.log(`App running at http://localhost:${port}`);
});
This creates a simple Express server and sets EJS as the view engine.
5. Adding Views
Navigate to the views/
folder and create a new file called index.ejs
. Inside this file, add the following:
<!DOCTYPE html>
<html>
<head>
<title>MVC Web App</title>
</head>
<body>
<h1><%= message %></h1>
</body>
</html>
Here, the <%= message %>
syntax is an EJS placeholder that will display data passed from the controller.
6. Adding a Controller
To follow the MVC principles, move your routing logic into a controller. Create a new file called homeController.js
inside the controllers/
folder:
exports.home = (req, res) => {
res.render('index', { message: 'Welcome to the MVC Web App!' });
};
Then, update your index.js
file to use this controller:
const homeController = require('./controllers/homeController');
app.get('/', homeController.home);
7. Running the App
To start your web app, use nodemon or Node.js:
- With nodemon:
npx nodemon index.js
- Without nodemon:
node index.js
Visit http://localhost:3000/ in your browser, and you should see your app running.
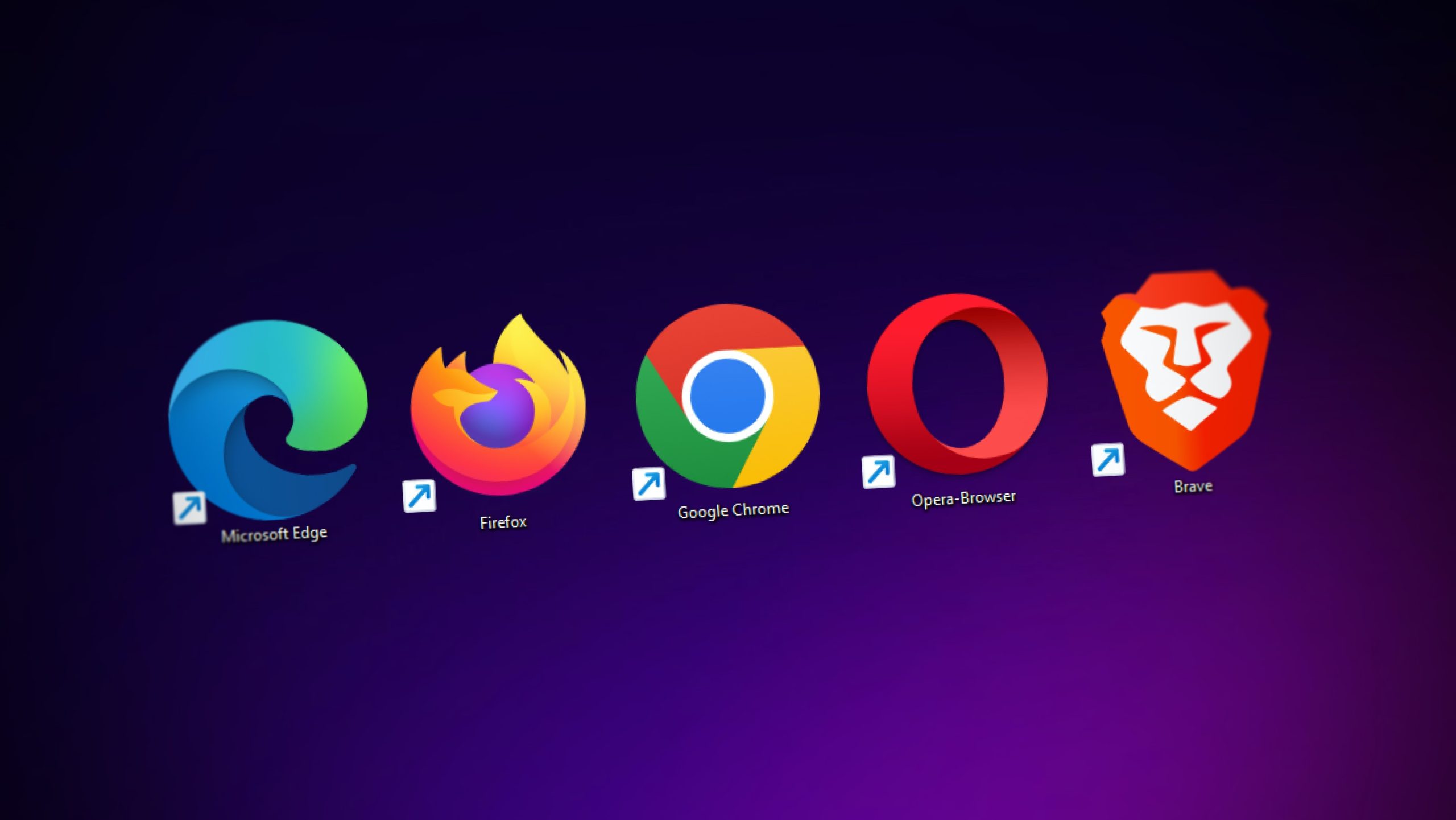
8. What’s Next?
Congratulations! You’ve built a basic MVC web app from scratch using only the terminal. From here, you can expand the app by:
- Adding new controllers and routes.
- Creating a database connection in the
models/
folder. - Incorporating CSS and JavaScript in the
public/
folder.
Building apps this way reinforces key concepts and trains you to troubleshoot effectively. Over time, GUI tools and advanced frameworks will become allies, but the core understanding you’ve built here will always serve you well.